In the previous article, we have successfully configured SonarQube with Jenkins and Jenkins can pull code from Gitlab, build the project automatically through Jenkinsfile. In this post, we will configure Docker with Jenkins.
Build the project with Maven
Updating the Jenkins file, we added two more stages, one is for building command project, another is for building others.
//git authorization ID
def git_auth = "7df0eb32-eb59-42cf-853a-58249fcede3b"
//git URL
def git_url = "git@192.168.1.20:infra_group/tensquare_back.git"
//image version
def tag = "latest"
//Harbor URL
def harbor_url = "192.168.1.13"
//image project name
def harbor_project = "tensquare"
//Harbor login credentials
def harbor_auth = "833d1a75-f3db-4aec-9cc4-75a77e423163"
node {
stage('check out code') {
checkout([$class: 'GitSCM', branches: [[name: '*/${branch}']],
doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [],
userRemoteConfigs: [[credentialsId: "${git_auth}", url:'git@192.168.1.20:infra_group/tensquare_back.git']]])
}
stage('code check') {
def scannerHome = tool 'sonarqube-scanner'
withSonarQubeEnv('sonarqube') {
sh """
cd ${project_name}
${scannerHome}/bin/sonar-scanner
"""
}
}
stage('build, install public subproject') {
sh "mvn -f tensquare_common clean install"
}
stage('build packages') {
sh "mvn -f ${project_name} clean package"
}
}
build again
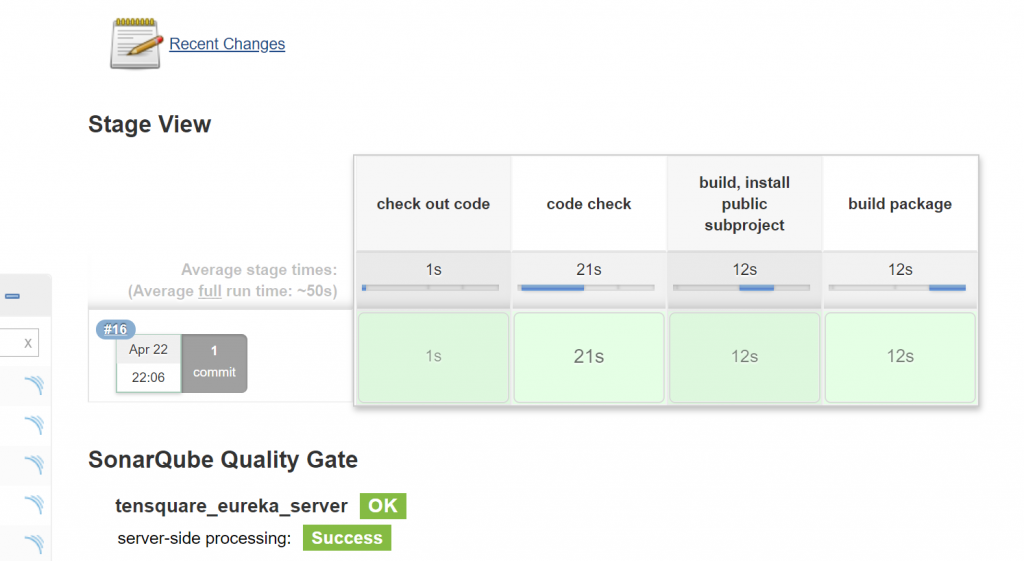
Build Docker image
Create Docker file in each project folder
#FROM java:8
FROM openjdk:8-jdk-alpine
ARG JAR_FILE
COPY ${JAR_FILE} app.jar
EXPOSE 10086
ENTRYPOINT ["java","-jar","/app.jar"]
Updating Jenkinsfile
//git authorization ID
def git_auth = "7df0eb32-eb59-42cf-853a-58249fcede3b"
//git URL
def git_url = "git@192.168.1.20:infra_group/tensquare_back.git"
//image version
def tag = "latest"
//Harbor URL
def harbor_url = "192.168.1.13"
//image project name
def harbor_project = "tensquare"
//Harbor login credentials
def harbor_auth = "833d1a75-f3db-4aec-9cc4-75a77e423163"
node {
stage('check out code') {
checkout([$class: 'GitSCM', branches: [[name: '*/${branch}']],
doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [],
userRemoteConfigs: [[credentialsId: "${git_auth}", url:'git@192.168.1.20:infra_group/tensquare_back.git']]])
}
stage('code check') {
def scannerHome = tool 'sonarqube-scanner'
withSonarQubeEnv('sonarqube') {
sh """
cd ${project_name}
${scannerHome}/bin/sonar-scanner
"""
}
}
stage('build, install public subproject') {
sh "mvn -f tensquare_common clean install"
}
stage('build package') {
sh "mvn -f ${project_name} clean package dockerfile:build"
//define image name and version
def imageName = "${project_name}:${tag}"
//add tag
sh "docker tag ${imageName} ${harbor_url}/${harbor_project}/${imageName}"
}
}
Before building, check the docker images on the Jenkins server. only a sonarqube image is existed.

build again and check docker images
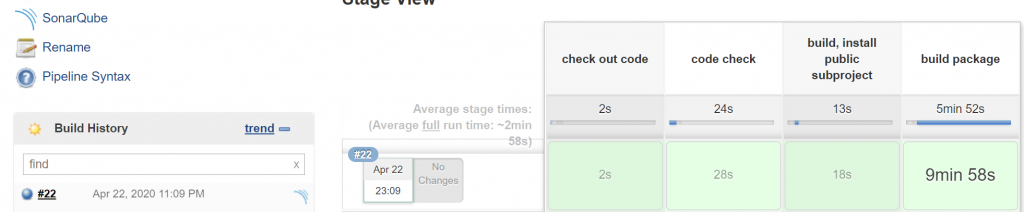

Push Docker image to Harbor
Updating Jenkinsfile, we added ‘upload docker image’ part.
//git authorization ID
def git_auth = "7df0eb32-eb59-42cf-853a-58249fcede3b"
//git URL
def git_url = "git@192.168.1.20:infra_group/tensquare_back.git"
//image version
def tag = "latest"
//Harbor URL
def harbor_url = "192.168.1.13"
//image project name
def harbor_project = "tensquare"
//Harbor login credentials
def harbor_auth = "0fb02305-eea7-492f-b395-43f2c0969d84"
node {
stage('check out code') {
checkout([$class: 'GitSCM', branches: [[name: '*/${branch}']],
doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [],
userRemoteConfigs: [[credentialsId: "${git_auth}", url:'git@192.168.1.20:infra_group/tensquare_back.git']]])
}
stage('code check') {
def scannerHome = tool 'sonarqube-scanner'
withSonarQubeEnv('sonarqube') {
sh """
cd ${project_name}
${scannerHome}/bin/sonar-scanner
"""
}
}
stage('build, install public tensquare_common') {
sh "mvn -f tensquare_common clean install"
}
stage('build package, Docker image, upload to Harbor') {
sh "mvn -f ${project_name} clean package dockerfile:build"
//define image name and version
def imageName = "${project_name}:${tag}"
//add tag
sh "docker tag ${imageName} ${harbor_url}/${harbor_project}/${imageName}"
//push docker image to Harbor
withCredentials([usernamePassword(credentialsId: "${harbor_auth}", passwordVariable: 'password', usernameVariable: 'username')]) {
//login Harbor
sh "docker login -u ${username} -p ${password} ${harbor_url}"
//upload docker image
sh "docker push ${harbor_url}/${harbor_project}/${imageName}"
sh "echo Docker image upload successfully"
}
}
}
build task again
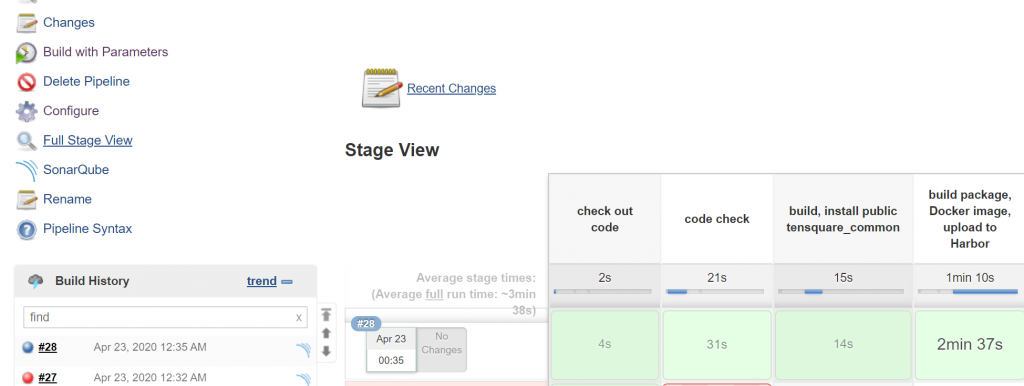
Check the result in Harbor
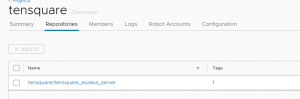
Conclusion
In this post, we builded project using Maven tool and created Docker image through Dockerfile, and added this two parts in Jenkinsfile. Jenkins is able to check which part are changed.